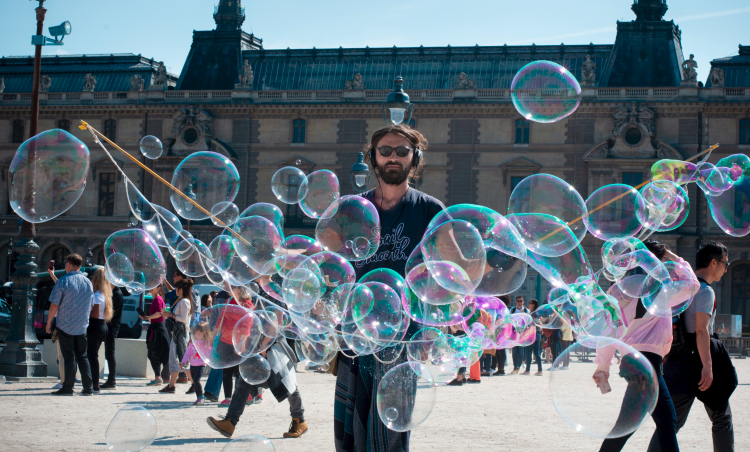
Can you write a Bubble sort in ruby?
The interviewer usually asks sorting algorithm and mostly the bubble sorting methods in the their interview. As that is one of the most commonly asked questions in sorting algorithm. So, I thought to share that in my blog here.
Bubble Sort
The bubble sort makes the larger elements (“big bubble”) towards the end and the smaller elements (“small bubble”) towards the starting point until all the elements reach in their correct location. i.e in proper sorted order from Small to Big sort.
# A method to define the bubble sort
def bubble_sort(array)
# condition: When array has more than one element
if array.count > 1
swap = true
# Loop: Run until swap is true
while swap
swap = false
# Loop: Run loop with array counts and swap if
(array.length-1).times do |z|
if array[z] > array[z+1]
# swap if current number element is greater than next number element.
array[z], array[z+1] = array[z+1], array[z]
# as we we have current number element is greater than next number element
# so we updated more swap needed.
swap = true
end
end
end
# While loop will stop once array[z] > array[z+1] will be false
# then swap will become false line number 8.
end
array
end
print "Befor sort - #{[7,9,3,5,4,2,1]}"
print "\n"
print "After sort - #{bubble_sort([7,9,3,5,4,2,1])}"
print "\n"
When you will run the above code( Public Gist here). tath will Result as below:
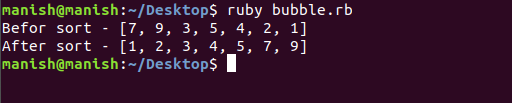
Thank you!